쥐수의 공부노트
백준 18258번 큐 2 본문
728x90
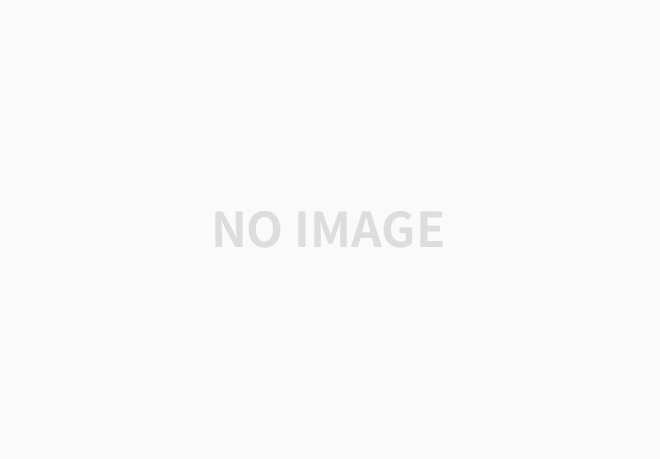
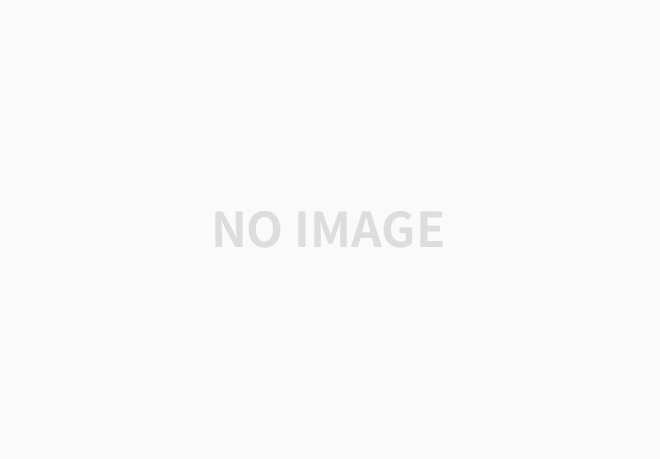
문제 자체는 바로 이전에 작성한 10845번 큐 문제와 동일하지만, 시간에 제한이 있다.
인터넷에는 Swift에서는 입출력이 느리기 때문에, 그것에 대한 문제도 있고, print에 문제가 있다고 나온다..
그래서 다른 사람의 풀이를 참고하여 진행하였고.. 나중에 시간이 나게 되면 다시 보도록 하자..
import Foundation
// 빠른 입력 FileIO
final class FileIO {
private var buffer:[UInt8]
private var index: Int
init(fileHandle: FileHandle = FileHandle.standardInput) {
buffer = Array(fileHandle.readDataToEndOfFile())+[UInt8(0)] // 인덱스 범위 넘어가는 것 방지
index = 0
}
@inline(__always) private func read() -> UInt8 {
defer { index += 1 }
return buffer.withUnsafeBufferPointer { $0[index] }
}
@inline(__always) func readInt() -> Int {
var sum = 0
var now = read()
var isPositive = true
while now == 10
|| now == 32 { now = read() } // 공백과 줄바꿈 무시
if now == 45{ isPositive.toggle(); now = read() } // 음수 처리
while now >= 48, now <= 57 {
sum = sum * 10 + Int(now-48)
now = read()
}
return sum * (isPositive ? 1:-1)
}
@inline(__always) func readString() -> Int {
var str = 0
var now = read()
while now == 10
|| now == 32 { now = read() } // 공백과 줄바꿈 무시
while now != 10
&& now != 32 && now != 0 {
str += Int(now)
now = read()
}
return str
}
}
struct Queue {
private var array: [Int] = []
private var index: Int = 0
var size: Int {
return array.count - index
}
var front: Int {
return self.isEmpty ? -1 : array[index]
}
var back: Int {
return self.isEmpty ? -1 : array.last!
}
var empty: Int {
return self.isEmpty ? 1 : 0
}
var isEmpty: Bool {
return array.count - index <= 0
}
mutating func push(_ element: Int) {
array.append(element)
}
mutating func pop() -> Int {
guard !self.isEmpty else {
return -1
}
let element = array[index]
index += 1
return element
}
}
let file = FileIO()
let n = file.readInt()
var queue: Queue = Queue()
var answer = ""
for _ in 0..<n {
let command = file.readString()
switch command {
case 448:
// push
queue.push(file.readInt())
case 335:
// pop
answer += "\(queue.pop())\n"
case 443:
// size
answer += "\(queue.size)\n"
case 559:
// empty
answer += "\(queue.empty)\n"
case 553:
// front
answer += "\(queue.front)\n"
case 401:
// back
answer += "\(queue.back)\n"
default:
continue
}
}
print(answer)
더보기
TMI : 출처 ( https://dev-mandos.tistory.com/191 )
728x90
'swift 알고리즘 > 큐, 덱' 카테고리의 다른 글
백준 5430번 AC (0) | 2023.06.16 |
---|---|
백준 1021번 회전하는 큐 (0) | 2023.06.16 |
백준 10866번 덱 (0) | 2023.06.14 |
백준 11866번 요세푸스 문제 0 (0) | 2023.06.13 |
백준 2164번 카드2 (1) | 2023.06.13 |